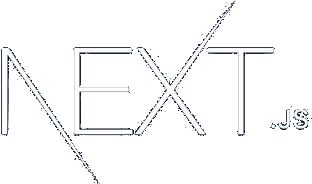
Next.js
Table of contents
Introduction
Currently my website is created with Next.js. The previous version was built using vanilla React, but I was experiencing issues every time I had to update all packages or even when I cloned the repository to a new machine. Both repositories can be found on my GitHub page, but the React version will probably disappear over time:
Web Server
The project is hosted at Vercel, which is a serverless solution. It can be linked to a repository so that every time the code is pushed, a deployment is initiated with automated testing. Suffice to say that this is much more efficient than doing this manually. A very long time ago I was used to doing this with FTP.
Setup
I will assume you already have Node, NPM and NPX installed. To set up a React app, you can enter the coommand below. The preferred name is given as parameter to this command:
1npx create-react-app my-app
For a Next.js app the command is almost identical:
1npx create-next-app@latest
However, the project name is not provided as a parameter and a couple of questions need to be answered:
1What is your project named? my-app
2Would you like to use TypeScript? No / Yes
3Would you like to use ESLint? No / Yes
4Would you like to use Tailwind CSS? No / Yes
5Would you like to use `src/` directory? No / Yes
6Would you like to use App Router ? (recommended) No / Yes
7Would you like to customize the default import alias (@/*)? No / Yes
I opted to not use TypeScript, I did install ESLint but I'm not yet using it. I also don't use Tailwind in this project. Furthermore I want to have all of my source code bundled in the 'src' directory, keeping the root directory clean. Of course I'm using the App Router which also was one of the reasons to migrate to Next.js. I left the default import alias as is.
Now you can start up your project, it will usually be available at https://localhost:3000. You can then start editing the 'app/page.js' file.
1npm run dev
API's
I used Supabase as my database, you could call this a DaaS, although I did replace it with JSON files stored locally. I might migrate to using my own API in the future. I have some past experience with Laravel, you can also find a tutorial about that here. Back then I planned to use it as a CMS, but in this case I just want to use it as an API. I'm not sure yet if I will use full-blown Laravel, I might opt for Lumen.
Another API that I'm using is SoundCloud to grab all of my albums and tracks and allow for those to be played on my site.
Some other data is pulled from a JSON file that is hosted within this repository as well as some data from a Markdown file. I'm still searching for a solution for all of my images, this will probably be hosted in the Laravel instance in the future, but for now it also is part of the repository. I will update this tutorial with some code examples of all three variants of fetching data as soon as possible.
Some useful resources: